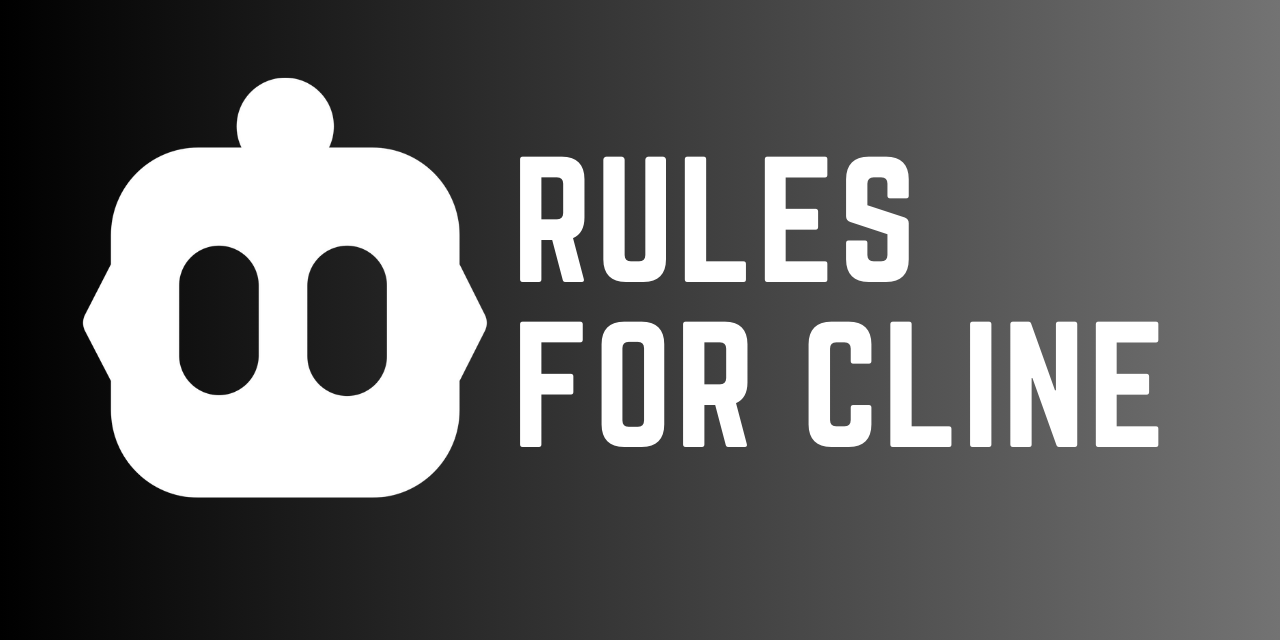
Creating Effective Rules for Cline AI: A Comprehensive Guide
Cline is an AI-powered coding assistant for VS Code that helps you build software by understanding your project and following specific rules. Setting up proper rules is essential for getting high-quality, consistent results from Cline. This guide will walk you through creating an effective .clinerules file to maximize Cline's performance on your projects.
## What is a .clinerules File?
A .clinerules
file is a project-specific configuration file that lives in your project's root directory. This file contains instructions that are automatically added to Cline's system prompt, ensuring it follows your project's standards and practices when generating or modifying code.
Unlike custom instructions (which are user-specific and apply globally), .clinerules files:
- Are version-controlled in your repository
- Apply to all team members working on the project
- Focus on project-specific standards and practices
- Serve as institutional knowledge about your project's rules
Creating Your .clinerules File
Basic Setup
- Create a file named
.clinerules
in your project's root directory - Use Markdown formatting for better organization
- Use clear sections with headings (##) to group related rules
- Be explicit and specific about your requirements
File Structure
Here's a template for an effective .clinerules file:
# Project Guidelines
## Project Overview
- Brief description of the project's purpose
- Key architectural patterns used
- Main dependencies and technology stack
## Code Structure
- File organization standards
- Naming conventions
- Module/component structure
## Code Style & Patterns
- Coding standards (indentation, formatting)
- Design patterns to follow
- Anti-patterns to avoid
- Error handling approach
## Documentation Requirements
- Documentation standards
- Comment style and requirements
- Required documentation files
## Testing Standards
- Testing frameworks used
- Test coverage requirements
- Types of tests needed (unit, integration, etc.)
## Performance Considerations
- Resource usage guidelines
- Optimization priorities
- Performance benchmarks to maintain
Example .clinerules File
Here's an example for a React/TypeScript project:
# React TypeScript Project Guidelines
## Project Overview
- This is a React SPA with TypeScript that provides an e-commerce dashboard
- Uses the Flux architecture pattern with Redux for state management
- Core dependencies: React 18, Redux Toolkit, TypeScript 5, Material UI 5
## Code Structure
- Use feature-based folder organization (/src/features/...)
- Follow atomic design patterns for components
- Keep components, hooks, and utilities in separate directories
- Use kebab-case for file names, PascalCase for component names
## Code Style & Patterns
- Use functional components with hooks, not class components
- Prefer composition over inheritance
- Use TypeScript interfaces for all props and state definitions
- Use the async/await pattern for all asynchronous operations
- Follow the container/presentational component pattern
- Format code using Prettier with project settings
## Error Handling
- Use try/catch for all async operations
- Implement error boundaries around major components
- Log errors using the logging utility in /src/utils/logging.ts
- Use toast notifications for user-friendly error messages
## Documentation Requirements
- Add JSDoc comments to all functions and components
- Include @param and @return tags in JSDoc comments
- Keep README.md up to date with project changes
- Use storybook for component documentation
## Testing Standards
- Write unit tests for all business logic using Jest
- Use React Testing Library for component tests
- Maintain at least 80% test coverage for business logic
- Write integration tests for critical user flows
## Performance Considerations
- Use React.memo for pure components
- Implement virtualization for long lists using react-window
- Keep bundle size under 250KB (minified + gzipped)
- Use code-splitting for large features
## Advanced Rule Configurations
Using a Rules Folder
For more complex projects, you can use a folder-based approach for better organization:
- Create a
.clinerules/
directory in your project root - Split rules into multiple Markdown files with logical grouping
- Use numeric prefixes for order (e.g.,
01-coding.md
,02-documentation.md
)
Example folder structure:
your-project/
.clinerules/ # Folder containing active rules
01-coding-standards.md
02-documentation.md
03-testing.md
current-sprint.md # Rules specific to current work
src/
...
Creating a Rules Bank
For teams working across multiple projects:
your-project/
.clinerules/ # Active rules - automatically applied
01-coding.md
client-a.md
clinerules-bank/ # Repository of available but inactive rules
clients/ # Client-specific rule sets
client-a.md
client-b.md
frameworks/ # Framework-specific rules
react.md
vue.md
project-types/ # Project type standards
api-service.md
frontend-app.md
...
Best Practices for Writing Effective Rules
Be specific and clear: Avoid ambiguity and provide concrete examples when possible
✅ Use TypeScript interfaces for component props ❌ Use strong typing
Prioritize importance: List the most critical rules first to ensure they get priority
Use hierarchy: Group related rules under clear headings to help Cline understand the structure
Explain the 'why': Briefly explain the reasoning behind important rules
- Always use React.memo for list item components to prevent unnecessary re-renders
Include examples: Demonstrate correct implementations for complex patterns
Example error handling:
try { await fetchData(); } catch (error) { logger.error('Data fetch failed', error); showErrorNotification('Failed to load data'); }
Be consistent with existing code: Ensure rules match your project's current practices
Define 'don'ts' clearly: Specify anti-patterns and what to avoid
Keep it reasonable: Don't overload with too many rules; focus on what matters most
Regular updates: Update rules as project practices evolve
Effective Prompts to Use with Cline
In addition to your .clinerules file, using effective prompts helps get better results:
Project Analysis Prompts
"Before writing code:
1. Analyze all code files thoroughly
2. Get full context
3. Write .MD implementation plan
4. Then implement code"
Quality Assurance Prompts
"DO NOT BE LAZY. DO NOT OMIT CODE."
"Rate confidence (1-10) before saving files, after saving, after rejections, and before task completion"
"List all assumptions and uncertainties you need to clear up before completing this task."
Architectural Prompts
"Check project files before suggesting structural or dependency changes"
"Ask 'stupid' questions like: are you sure this is the best way to implement this?"
## Sample .clinerules for Different Project Types
For a Backend Node.js API
# Node.js API Guidelines
## Project Structure
- Use a layered architecture (routes → controllers → services → repositories)
- Follow RESTful design principles
- Group routes by resource type
- Place shared middleware in /src/middleware
- Place DB models in /src/models
## Coding Standards
- Use ES6 module syntax (import/export)
- Use async/await for all asynchronous operations
- Implement proper error handling with try/catch
- Use meaningful variable and function names
## API Design
- Use plural nouns for resource endpoints (/users not /user)
- Follow HTTP method semantics (GET, POST, PUT, DELETE)
- Return appropriate HTTP status codes
- Structure response objects consistently
- Include pagination for list endpoints
## Testing Requirements
- Write unit tests for all business logic
- Write integration tests for API endpoints
- Use Jest and Supertest for testing
- Test both success and error cases
## Error Handling & Logging
- Use the centralized error handler in /src/middleware/error-handler.js
- Log all errors with appropriate contexts
- Return standardized error responses with useful messages
- Never expose sensitive information in error responses
## Security Practices
- Validate and sanitize all user inputs
- Use parameterized queries for database operations
- Implement proper authentication and authorization
- Set appropriate security headers
For a Frontend React Application
# React Frontend Guidelines
## Component Structure
- Use functional components with hooks
- Split large components into smaller ones
- Keep components focused on a single responsibility
- Use the Container/Presenter pattern for complex components
## State Management
- Use React Context for global UI state
- Use Redux for complex application state
- Keep Redux actions and reducers in feature-focused files
- Use Redux Toolkit to reduce boilerplate
## Styling Approach
- Use CSS Modules for component styling
- Follow BEM naming convention for CSS classes
- Maintain a consistent color palette from /src/styles/variables.scss
- Ensure all components are responsive
## Performance Optimization
- Use React.memo for pure components
- Implement virtualization for long lists
- Use React.lazy and Suspense for code-splitting
- Optimize images and assets
## Accessibility Standards
- All interactive elements must be keyboard accessible
- Use semantic HTML elements
- Include aria attributes where appropriate
- Maintain a minimum contrast ratio of 4.5:1
- Ensure focus states are visible
## Testing Requirements
- Unit test all components
- Test component behavior, not implementation
- Test all user interactions
- Use mock services for API calls
## Conclusion
Creating an effective .clinerules file is a powerful way to guide Cline AI in generating high-quality, consistent code that follows your project's standards and best practices. The key is to be clear, specific, and comprehensive while focusing on what matters most for your project.
Start with a basic structure, customize it to your project's needs, and refine it over time based on results. Remember that the .clinerules file is part of your project's documentation and should evolve as your project and team grow.
By investing time in creating good rules, you'll make Cline a more effective coding partner, helping your team build better software more efficiently.
Comments (0)
No comments yet. Be the first to comment!
You must be signed in to comment.